home
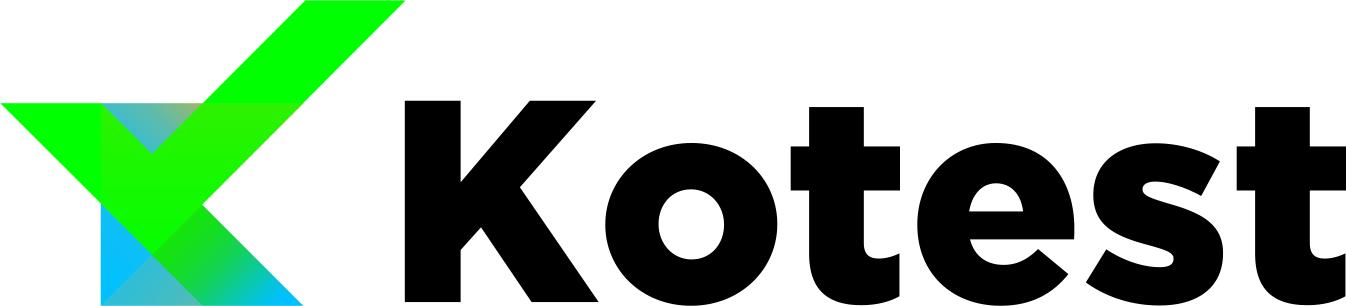
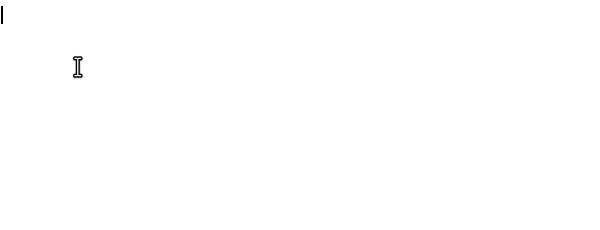
Kotest is a flexible and comprehensive testing project for Kotlin with multiplatform support.
For latest updates see Changelog.
See our quick start guide to get up and running.
Community​
- Stack Overflow (don't forget to use the tag "kotest".)
- Kotest channel in the Kotlin Slack (get an invite here)
- Contribute
Test with Style​
Write simple and beautiful tests with the StringSpec
style:
class MyTests : StringSpec({
"length should return size of string" {
"hello".length shouldBe 5
}
"startsWith should test for a prefix" {
"world" should startWith("wor")
}
})
Kotest comes with several testing styles so you can choose one that fits your needs.
Multitude of Matchers​
Use over 300 provided matchers to test assertions on many different types:
"substring".shouldContain("str")
user.email.shouldBeLowerCase()
myImageFile.shouldHaveExtension(".jpg")
cityMap.shouldContainKey("London")
The withClue
and asClue
helpers can add extra context to assertions so failures are self explanatory:
withClue("Name should be present") { user.name shouldNotBe null }
data class HttpResponse(val status: Int, body: String)
val response = HttpResponse(200, "the content")
response.asClue {
it.status shouldBe 200
it.body shouldBe "the content"
}
Nesting is allowed in both cases and will show all available clues.
Matchers are extension methods and so your IDE will auto complete. See the full list of matchers or write your own.
Let the Computer Generate Your Test Data​
Use property based testing to test your code with automatically generated test data:
class PropertyExample: StringSpec({
"String size" {
checkAll<String, String> { a, b ->
(a + b) shouldHaveLength a.length + b.length
}
}
})
Check all the Tricky Cases With Data Driven Testing​
Handle even an enormous amount of input parameter combinations easily with data driven tests:
class StringSpecExample : StringSpec({
"maximum of two numbers" {
forAll(
row(1, 5, 5),
row(1, 0, 1),
row(0, 0, 0)
) { a, b, max ->
Math.max(a, b) shouldBe max
}
}
})
Test Exceptions​
Testing for exceptions is easy with Kotest:
val exception = shouldThrow<IllegalAccessException> {
// code in here that you expect to throw an IllegalAccessException
}
exception.message should startWith("Something went wrong")
Fine Tune Test Execution​
You can specify the number of invocations, parallelism, and a timeout for each test or for all tests.
And you can group tests by tags or disable them conditionally.
All you need is config
:
class MySpec : StringSpec({
"should use config".config(timeout = 2.seconds, invocations = 10, threads = 2, tags = setOf(Database, Linux)) {
// test here
}
})